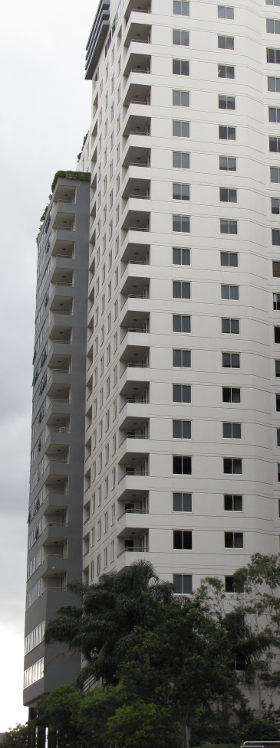
Sydney Apartment Block
Apartments. They are widely marketed as a preferable alternative to the traditional house and garden. And there
are many advantages. But the reality is there are many, many disadvantages, too. The disadvantages will be the primary focus of this article.
Advantages of Apartment Living
Let’s start with the advantages of apartment living to keep the article, to some extent, balanced.
Firstly many apartments are quite modern being a modern phenomena in many places. It is quite appealing to be living in a new place with fresh paint and new appliances.
Because apartment blocks can be quite densely populated they can often afford to be positioned near amenities such as public transport or shopping centres.
Disadvantages
Noise
Apartment blocks are noisy. You cannot escape this. Ever. Why? Because you will always get a neighbour, either upstairs, or downstairs, or to your left, or to your right, that has their powerful sub-woofer pumping away, or does the vacuuming or washing at 11pm at night, or has super-noisy coitus.
Modern apartments have thick walls – and even concrete floors – but they are not always thick enough – especially for modern powerful sub-woofers.
Older apartments or maisonettes – as are popular in overcrowded London – have notoriously thin wooden floors and you can hear everything going on below and above.
Garbage
In the UK, at least, unwanted material can be separated into recycling and garbage. In an individual household that is not really a problem. But in an apartment block you are typically given one large garbage bin and one large recycling bin for the entire block.
This shouldn’t be a problem: however apartment blocks are often full of renters – many of whom are from cultures somewhat incompatible with the local. These people flat don’t understand or care about the rules for recycling that are plastered all over the bin. They throw dirty nappies, plastic bags, and other unrecyclable items into the recycling bin.
And then the council refuses to empty the overflowing garbage tip the recycling bin has become. And the council then writes to all the apartment residents blaming them for failing to obey the recycling rules.
Apartment blocks allow a level of anonymity when it comes to garbage and this lack of accountability means everybody in the block suffers.
Parking and Access
If your apartment block is gated you can expect to be harassed by people you never met pressing your buzzer hoping you’ll let them in. Tradesmen will just press any old button in an attempt to turn up for an appointment for someone else.
Often you will have an allocated space. But you can have fun dealing with a neighbour that parks badly leaving you with little room in your own space.
In the UK one has to watch out for apartment blocks that have no visitor parking. Expecting your friends to pay for parking nearby? Doesn’t seem fair, now, does it.
Quality with Age
Some apartment blocks are built very poorly. I had experience living in a Sydney apartment block that was a 30 story tower. I was on the 6th floor but, at only 7 years old, had cracks in the concrete walls and the floor was sloping. In Australia, especially, one must be vary careful with build quality as many large apartment-makers in that country have a reputation for poor quality.
Recent Comments